Use our API to create your own front-end apps.
In our documentation, you can use our different endpoints to fetch data and create your customs front-end.
You only need to make the corresponding HTTP request to the API endpoint using the correct PATH give on the help reference:
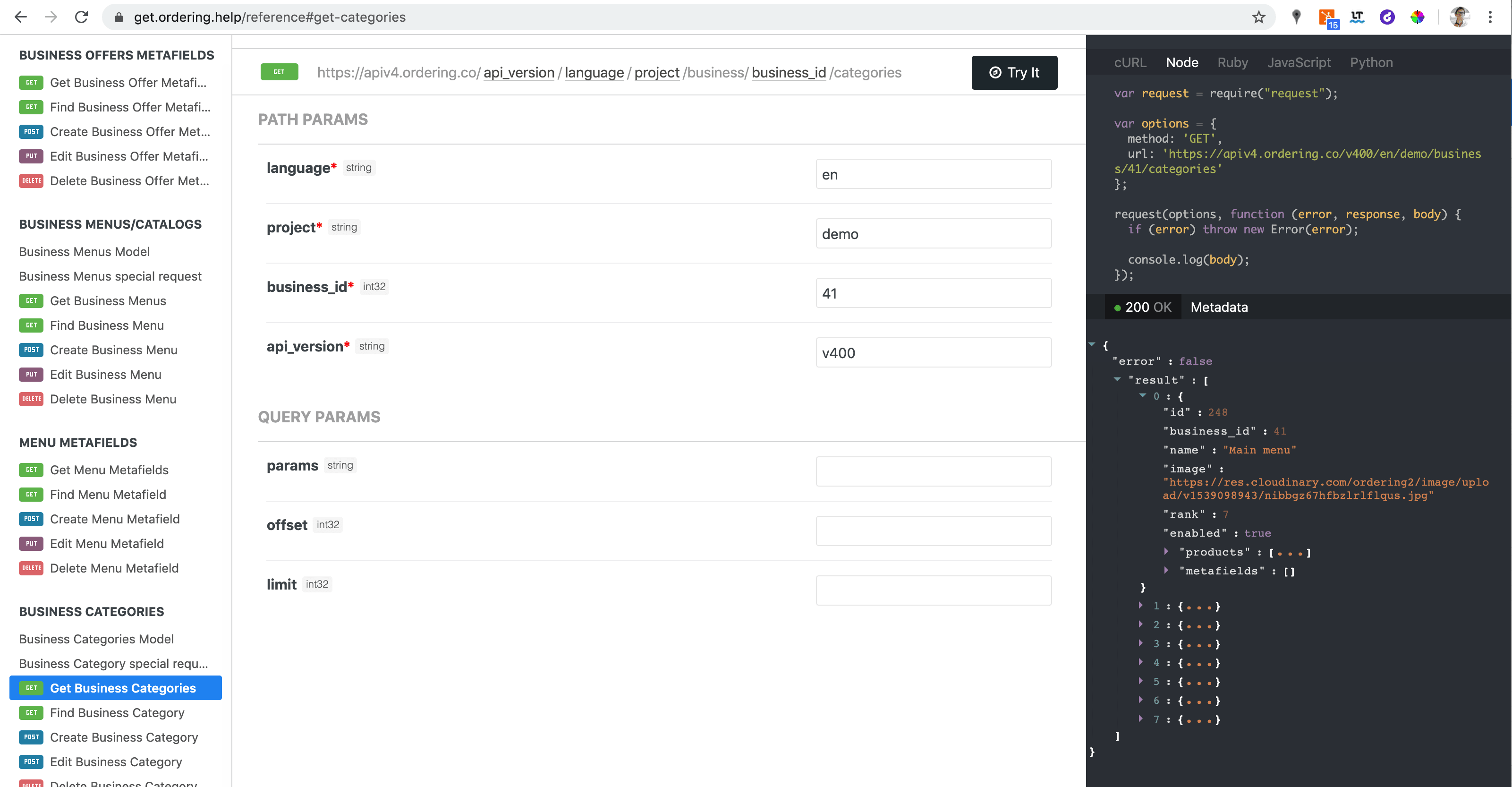
Fetch the JSON response and use it to build your app. Check the example code bellow:
import React, { Component } from 'react';
import axios from 'axios';
import SearchBar from './SearchBar';
import ProductList from './products/ProductList';
class Store extends Component {
state = { categories: [], searchProducts: '' };
componentDidMount() {
this.fetchCategories();
}
async fetchCategories() {
const orderingApi = axios.create({
baseURL: 'https://apiv4.ordering.co/',
headers: {
'x-api-key': 'TYjvt1hGDxdhQOZtQ1kn9kRXYXHIn1ljGdblGYx07rSscGBL0zGqq63Wzx9MOQyQJ'
}
});
const response = await orderingApi.get('/v400/en/demo/business/41');
this.setState({ categories: response.data.result.categories });
}
renderCategoriesList(searchProducts) {
return this.state.categories.map(category => {
return <ProductList category={category} searchProducts={this.state.searchProducts} key={category.id} />;
});
}
onInputChange = (term) => {
this.props.searchProductsByName(term);
}
render() {
return (
<div>
<SearchBar onInputChange={this.onInputChange} />
<div className="ui segment">
{this.renderCategoriesList(this.props.searchProducts)}
</div>
</div>
)
}
}
export default Store;
//store.component.html
<div>
<div class="categories card m-2" *ngFor="let categorie of categories">
<div class="title">
<h3>{{ categorie.name }}</h3>
</div>
<!-- ProductList -->
<div class="container-fluid">
<div
class="productContainer row"
*ngFor="let product of categorie.products"
>
<div class="productImage col-2">
<img src="{{ product.images }}" alt="" />
</div>
<div class="productName col-5">
{{ product.name }}
</div>
<div class="productButton col-5">
<button class="btn btn-success">Add to cart</button>
</div>
</div>
</div>
<!-- End of ProductList -->
</div>
</div>
//store.component.ts
import { Component, OnInit } from "@angular/core";
@Component({
selector: "app-store",
templateUrl: "./store.component.html",
styleUrls: ["./store.component.css"]
})
export class StoreComponent implements OnInit {
categories = [];
constructor() {}
async fetchData() {
const response = await fetch(
"https://apiv4.ordering.co/v400/en/demo/business/41/categories"
);
const data = await response.json();
const categories = data.result;
this.categories = categories;
console.log(this.categories);
}
ngOnInit(): void {
this.fetchData();
}
}
<template>
<div>
<div
class="categories card m-2"
v-for="(categorie, index) in this.categories"
v-bind:key="index"
>
<div class="title">
<h3>{{ categorie.name }}</h3>
</div>
<ProductList v-bind:products="categorie.products" />
</div>
</div>
</template>
<script>
import axios from 'axios';
import ProductList from './products/ProductList.vue';
export default {
name: 'Store',
components: {
ProductList
},
data: function() {
return {
categories: {}
};
},
methods: {
async fetchData() {
const categories = await axios.get(
'https://apiv4.ordering.co/v400/en/demo/business/41/categories'
);
this.categories = categories.data.result;
}
},
beforeMount() {
this.fetchData();
}
};
</script>
<style scoped>
.title {
margin: 30px;
border-bottom: 1px solid rgba(0, 0, 0, 0.2);
}
</style>
To get something like this:
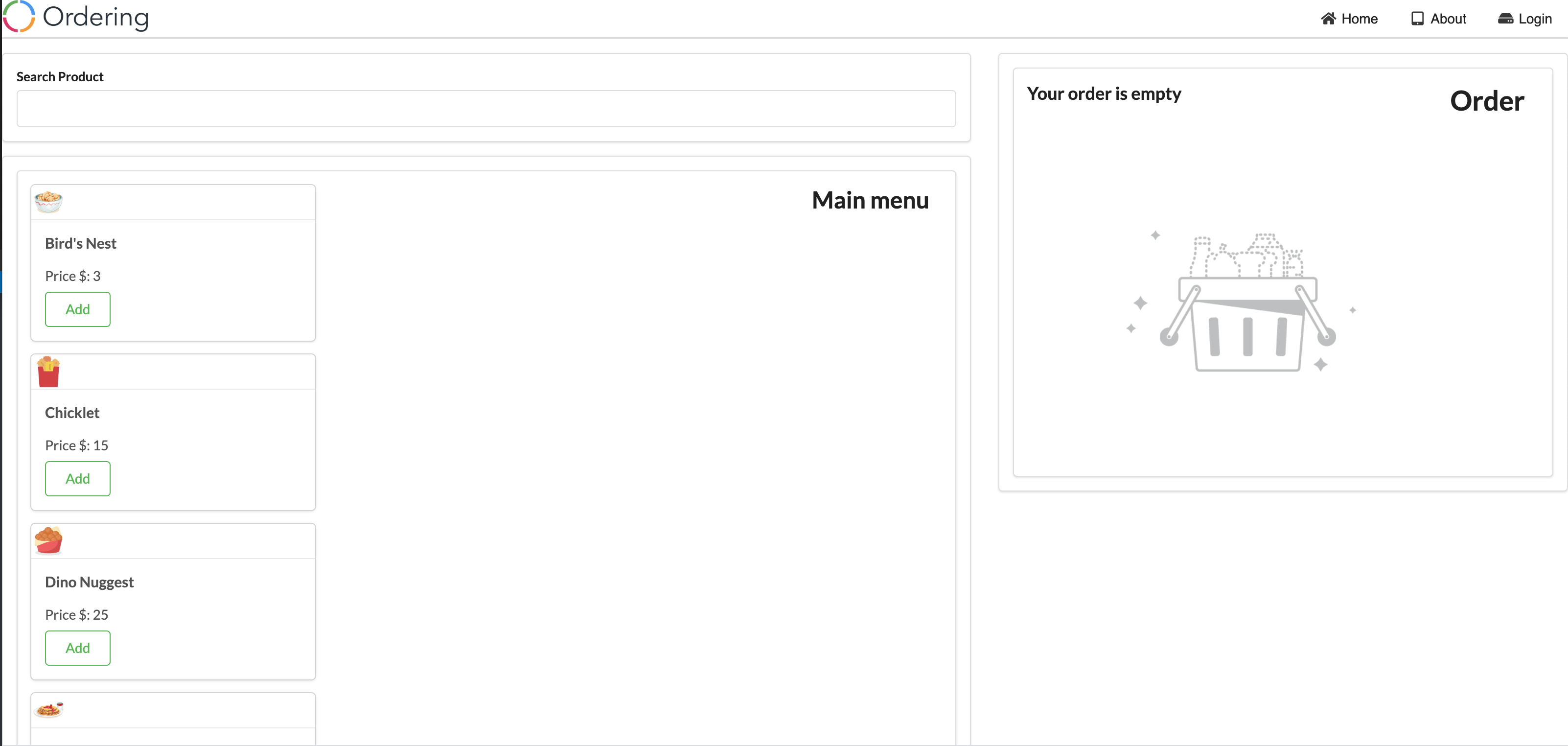
All our API power can be on your hands, so give it a check to our documentation on https://get.ordering.help/reference
If you need anything else from your Ordering Team, just let us know 👍
Have a Happy Ordering
Have a Happy Ordering
Carlos Fuentes
Support TeamUpdated about 4 years ago