Used to authorize users with Facebook Login.
Current allowed platforms
- Apple
Platform | Body params |
---|---|
access_token | |
access_token | |
apple | - name: Optional (Get from apple on the first login with apple) - lastname: Optional (Get from apple on the first login with apple) - code: Get from apple login frontend |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Apple Login</title>
<script type="text/javascript" src="https://appleid.cdn-apple.com/appleauth/static/jsapi/appleid/1/en_US/appleid.auth.js"></script>
</head>
<body>
<a href="#" onclick="onSignIn();">Sign in with apple id</a>
<script type="text/javascript">
AppleID.auth.init({
clientId : 'YOUR_CLIENT_ID',
scope : 'name email',
redirectURI : 'https://e6b6ae1cf48a.ngrok.io/applelogin.html',
// state : '[STATE]',
// nonce : '[NONCE]',
usePopup : true //or false defaults to false
});
async function onSignIn() {
try {
const data = await AppleID.auth.signIn()
console.log(data)
} catch ( error ) {
console.log(error)
}
}
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta name="google-signin-client_id" content="67******4880-si**********ncrmbv84.apps.googleusercontent.com">
<title>Google Login</title>
<script src="https://apis.google.com/js/platform.js" async defer></script>
</head>
<body>
<div class="g-signin2" data-onsuccess="onSignIn"></div>
<a href="#" onclick="signOut();">Sign out</a>
<script>
function onSignIn(googleUser) {
var id_token = googleUser.getAuthResponse().id_token;
console.log(id_token)
}
function signOut() {
var auth2 = gapi.auth2.getAuthInstance();
auth2.signOut().then(function () {
console.log('User signed out.');
});
}
</script>
</body>
</html>
Access_token Facebook
This access token is the one that RETURNS facebook when you log in with it and sends it to the API to log in a user if it exists or register and login if it does not exist. More information about getting an Access token here
Access_token google
This access token is the one that RETURNS google when you log in with it and sends it to the API to log in a user if it exists or register and login if it does not exist. More information about getting an Access token here
Code Apple
This code is the one that RETURNS apple when you log in with it and sends it to the API to log in a user if it exists or register and login if it does not exist. More information about getting a Code here
Functionality
Logging in with Social for applications is a quick and convenient way to create accounts and log in to your application on various platforms.
Register user:
Logging in with Socials allows people to register quickly and easily in your application without having to set a password. The simplicity and convenience of the experience translates into greater conversion. Once someone creates an account on a platform, you can log in to the application, often with a single click.
Login to different platforms:
Logging in with Social is available on most mobile and computer application platforms. After creating an account with Social on a platform, people can log in quickly and easily in any version of the application on other platforms. The user identification does not vary, so you can resume the experience with the application where you left off. The login with Social is available for iOS apps, Android apps, Websites, Windows Phone Apps, applications for computers and devices, such as Smart TVs and Internet of things devices.
User Levels:
- Only customers can Register with Social.
- If any other user level has an account already registered and the email is the same used on Social, then Social login can be enabled for that account, it is enabled automatically and is related to the email previously registered.
Facebook Login Requirements
Facebook login setup is required.
To check if Facebook configuration exists use Get Configurations, the configurations that Facebook use arefacebook_id
andfacebook_secret
. If facebook configuration does not exist, it must be created, to add values, see Create configuration.
Values can be obtained from Facebook developers website, for reference please check Facebook configurations
Google Login Requirements
The configurations that Google use is
google_login_client_id
which id the client id of the google account . If Google configuration does not exist, it must be created, to add values, see Create configuration.
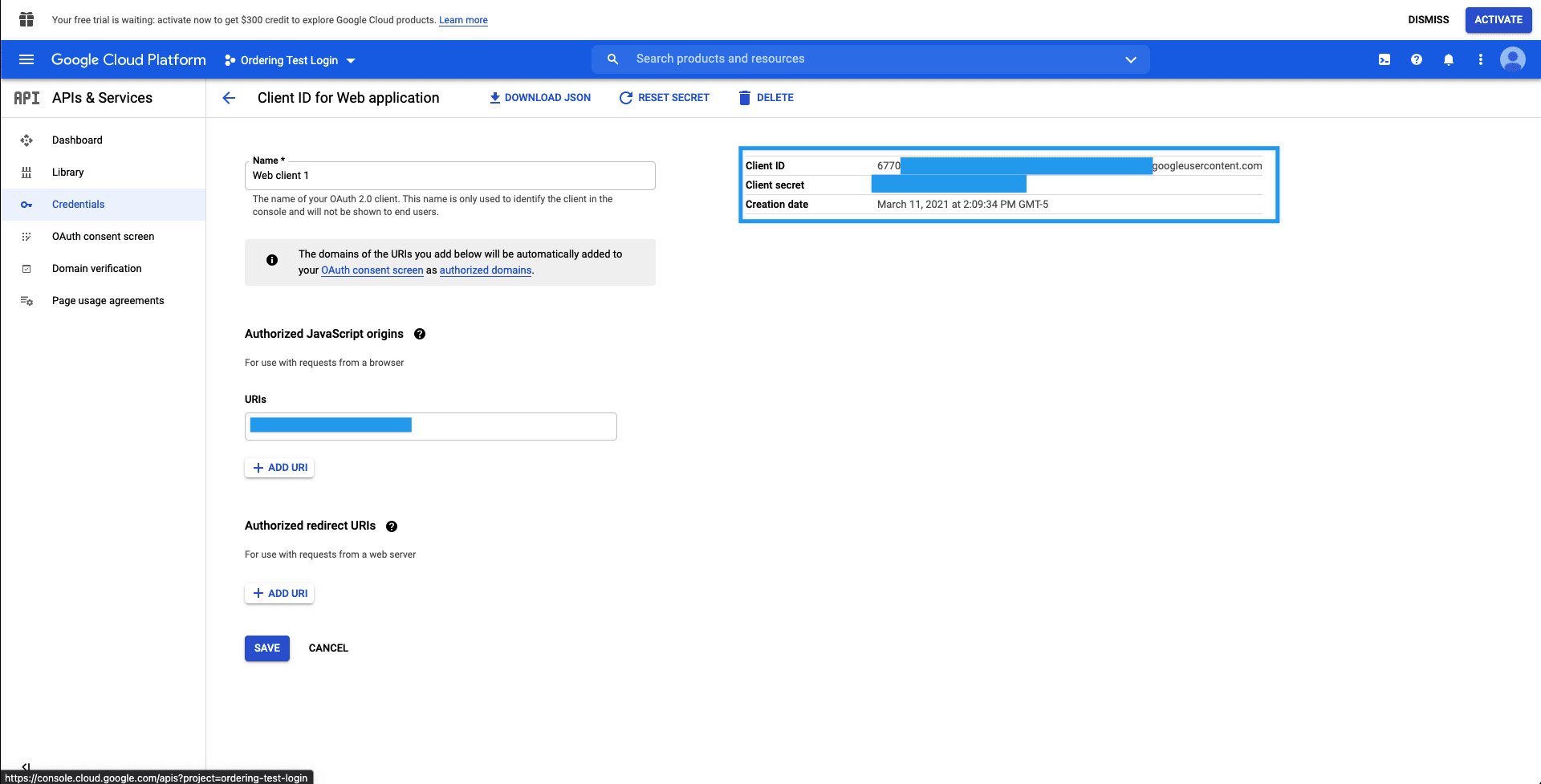
Apple Login Requirements
Apple login setup is required.
The configurations that Facebook use are:
apple_team_id
: Apple team id
apple_login_private_key_id
: Apple key id get from (https://developer.apple.com/account/resources/authkeys/list)
apple_login_private_key
: Apple key download at create time from (https://developer.apple.com/account/resources/authkeys/add)
apple_login_client_id
: Apple service indentifier from (https://developer.apple.com/account/resources/identifiers/list/serviceId)If Apple configuration does not exist, it must be created, to add values, see Create configuration.