The special data types is data that needs to have a specific structure to be able to be handled correctly in the API. For the most part, this data is in JSON, which is why this structure must be followed.
Schedules
The schedules are data that is used in the businesses, menus and delivery areas. These are sent as JSON with the following structure:
[
{
"enabled":true,
"lapses":[
{
"open":{
"hour":0,
"minute":0,
},
"close":{
"hour":23,
"minute":59,
}
},
...
]
},
...
]
- Each object represents a day, it must have 7 days, being Sunday the first day and Saturday the last day.
enabled
represents if that day is available.lapses
represents the times when the business is open, it can have several lapses for multiple time windows for that day.open
andclose
, have attributes ofhour
andminute
, which are the hours and minutes of the opening and closing times for the lapse respectively.hour
is represented in 24-hour format, from0
for12:00 AM
to23
for11:00 PM
andminute
from0
to59
.
The full example of a business schedule
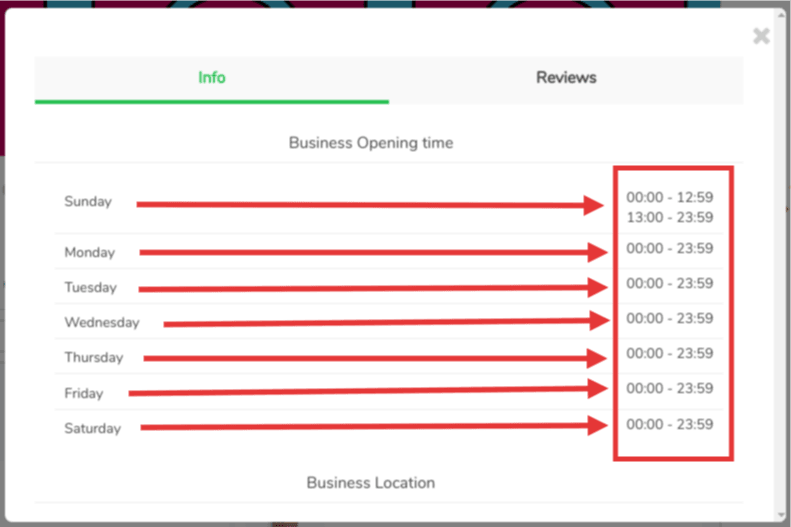
[
{ //sunday
"enabled": true,
"lapses": [
{
"open": {
"hour": 0,
"minute": 0
},
"close": {
"hour": 12,
"minute": 59
}
},
{
"open": {
"hour": 13,
"minute": 0
},
"close": {
"hour": 23,
"minute": 59
}
}
]
},
{ //monday
"enabled": true,
"lapses": [
{
"open": {
"hour": 0,
"minute": 0
},
"close": {
"hour": 23,
"minute": 59
}
}
]
},
{ //tuesday
"enabled": true,
"lapses": [
{
"open": {
"hour": 0,
"minute": 0
},
"close": {
"hour": 23,
"minute": 59
}
}
]
},
{ //wednesday
"enabled": true,
"lapses": [
{
"open": {
"hour": 0,
"minute": 0
},
"close": {
"hour": 23,
"minute": 59
}
}
]
},
{ //thursday
"enabled": true,
"lapses": [
{
"open": {
"hour": 0,
"minute": 0
},
"close": {
"hour": 23,
"minute": 59
}
}
]
},
{ //friday
"enabled": true,
"lapses": [
{
"open": {
"hour": 0,
"minute": 0
},
"close": {
"hour": 23,
"minute": 59
}
}
]
},
{ //saturday
"enabled": true,
"lapses": [
{
"open": {
"hour": 0,
"minute": 0
},
"close": {
"hour": 23,
"minute": 59
}
}
]
}
]
Order products
To create an order you must send the products in JSON format, they should be sent following
this structure:
[
{
"id": 1,
"quantity": 1,
"comment": "Example comment",
"ingredients": [1,2,..],
"options": [
{
"id": 1,
"suboptions": [1,2,3...]
},
...
]
},
...
]
[
{
"id": 1,
"quantity": 1,
"comment": "Example comment",
"ingredients": [1,2,..],
"options": [
{
"id": 1,
"suboptions": [
{
id: 1,
position: "whole",
quantity: 1,
},
...
]
},
...
]
},
...
]
- Each object represents a product, the products must belong to the business of the order.
id
is the product identifier.quantity
is the quantity ordered for this product in the order.comment
is the comment for special requests.ingredients
is an array of the ingredient identifier.options
are the options chosen by the customer of this product.id
is the option identifier.suboptions
is an array of the suboption identifier.
NEW FEATURE
suboptions
now also accepts a strict JSON that contains the position of the subption (left, whole, right). the price of the middle suboption depends on 'half_price' attribute of the option.
[
{"id":1309,
"code":"JmVLFm",
"quantity":1,
"options":[
{
"id":53,
"suboptions":[
174
]
},
{
"id":54,
"suboptions":[
175,
176
]
},
{
"id":55,
"suboptions":[
178
]
},
{
"id":71,
"suboptions":[
238
]
}
],
"ingredients":[
],
"comment":"With Special sauce"
}
]
Locations
The location is used in businesses, users, addresses and drivers, they are represented in JSON format with the following structure:
{
"lat": 0,
"lng": 0
}
lat
is the latitude of the position.lng
is the longitude of the position.
Polygons
The polygons is used in the delivery areas, they are represented in JSON format and have the following structure:
[
{
"lat": 0,
"lng": 0
},
...
]
For the polygon to be valid, you must have at least 3 locations
Example
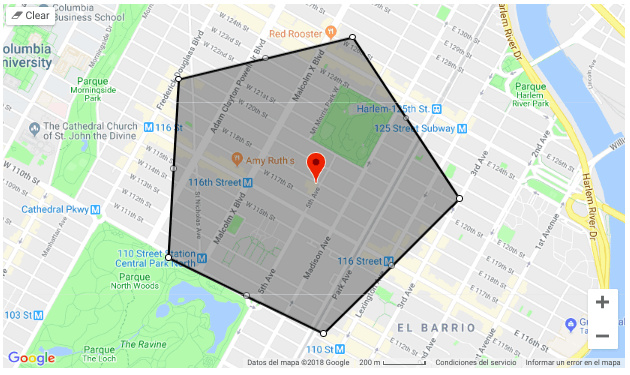
[
{
"lat":40.80651051083527,
"lng":-73.95360356106994
},
{
"lat":40.80832956284492,
"lng":-73.94364720120666
},
{
"lat":40.8013234583049,
"lng":-73.93754596573359
},
{
"lat":40.795475710711585,
"lng":-73.94531364304072
},
{
"lat":40.79875701029246,
"lng":-73.95419711929804
}
]
Radios
The radio is used in the delivery areas, they are represented in JSON format and have the following structure:
{
"center": {
"lat": 0,
"lng": 0
},
"radio": 10
}
center
is the location of the center for the radio.radio
is the maximum distance from the center in kilometers.
Customers
Customers data must be sent in JSON format, this should be sent following this structure:
{
"id": 1,
"name": "Demo",
"photo": null,
"lastname": "test1",
"email": "[email protected]",
"dropdown_option_id": null,
"address": "5th Ave, New York, NY, USA",
"address_notes": "Special address instrution example",
"zipcode": null,
"cellphone": "2332223",
"phone": "23322235546",
"location": {
"lat": 40.7314123,
"lng": -73.9969848
}
"map_data": {
"library": "google"
"place_id": "ChIJgUbEo8cfqokR5lP9_Wh_DaM"
}
}
Map data
map_data must be sent as null or object in the customer data , this should be sent following this structure:
{
"library": "google",
"place_id": "ChIJgUbEo8cfqokR5lP9_Wh_DaM"
}
Note: The id of the customer can be sent with a value of
-1
for non-registered users